I’m convinced that implicitness leads to a higher WTF per Minute (WTFPM) in the long run.
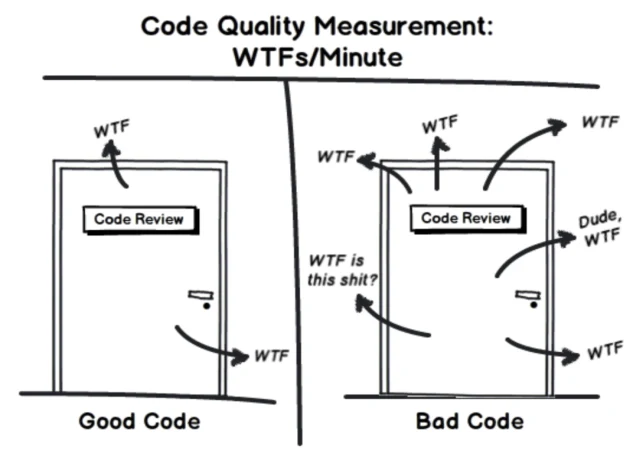
Implicitness requires the coder to remember hidden details instead of having them clearly defined in the code. For example, can you tell me why we’re adding “10” here?
// Implicit (bad)function calculateSalary(a, b) { return a + b + 10; // a?? b?? WTF is 10?}
Exactly, that was the point. No one can tell what “10” represents except the person who wrote it, and that’s awful in my opinion. If you have a team of engineers working on a project, how can anyone possibly navigate the codebase with such ambiguity?
Instead of implicitly adding “10”, we can prevent future headaches by simply creating a clear variable for the bonus. In my view, relying too heavily on implicit behavior can cause confusion and make managing the codebase more difficult.
// Explicit (good)function calculateSalary(cash, equity) { const bonus = 10; // Explicit bonus variable return cash + equity + bonus;}
Tim Peters’ second point in The Zen of Python states, “Explicit is better than implicit,” and I couldn’t agree more.
Imagine a function that grants access to different parts of a system based on a user’s role. In this example, implicit behavior leads to confusion about which roles have which permissions, making the code difficult to maintain.
// Implicit (bad)function grantAccess(user) { if (user.role === 'admin') { return ['read', 'write', 'delete']; // Full access }
if (user.role === 'editor') { return ['read', 'write']; // Editors can't delete }
if (user.role === 'viewer') { return ['read']; // View-only access }
// Implicit fallback return ['none']; // WTF is 'none'?}
function showAccessControls(user) { const permissions = grantAccess(user);
if (permissions.includes('read')) { console.log("User has read access."); } if (permissions.includes('write')) { console.log("User has write access."); } if (permissions.includes('delete')) { console.log("User has delete access."); }}
// Example usageconst user1 = { name: "Alice", role: "admin" };const user2 = { name: "Bob", role: "viewer" };const user3 = { name: "Charlie", role: "guest" }; // Implicit 'none' fallback
showAccessControls(user1); // Has all accessshowAccessControls(user2); // Read-only accessshowAccessControls(user3); // No access, but why?
The access control is handled with hard-coded roles and magic strings like ‘none’, making it unclear and unmanageable as more roles are added. Permissions are scattered throughout the code, leading to confusion and maintenance issues for future developers.
// Explicit (good)const ROLE_PERMISSIONS = { admin: ['read', 'write', 'delete'], editor: ['read', 'write'], viewer: ['read'], guest: [] // Explicitly defining guest permissions};
function grantAccess(user) { const permissions = ROLE_PERMISSIONS[user.role] || ROLE_PERMISSIONS['guest']; return permissions;}
function showAccessControls(user) { const permissions = grantAccess(user);
if (permissions.includes('read')) { console.log(`${user.name} has read access.`); } if (permissions.includes('write')) { console.log(`${user.name} has write access.`); } if (permissions.includes('delete')) { console.log(`${user.name} has delete access.`); } if (permissions.length === 0) { console.log(`${user.name} has no access.`); }}
// Example usageconst user1 = { name: 'Alice', role: 'admin' };const user2 = { name: 'Bob', role: 'viewer' };const user3 = { name: 'Charlie', role: 'guest' };
showAccessControls(user1); // Alice has read, write, and delete access.showAccessControls(user2); // Bob has read access.showAccessControls(user3); // Charlie has no access.
Why Explicit is Better
-
Explicit Mapping: The
ROLE_PERMISSIONS
object clearly defines each role and its corresponding permissions. -
Clarity: By making permissions explicit, there’s no need for magic strings or conditionals scattered across the codebase.
-
Maintainability: Future roles or changes to permissions can be easily updated in one place.
In my opinion, “Explicit is better than implicit” is about choosing clear, direct code over concise but potentially ambiguous alternatives. This principle puts readability and maintainability first, even if it means writing slightly more verbose code.
By being explicit, the intentions behind the code are obvious, making it easier for any developer to understand and work with the codebase.